If you’re new to blockchain development, and just starting to interact with contracts, one subject that may come up is how to decode a transaction hash on the polygon network. Luckily, web3.js is here to help! web3.js is a set of libraries that let you communicate with Ethereum nodes using HTTP, IPC or WebSocket. With web3.js, you can read and write data on the blockchain, send transactions, and interact with smart contracts. In this tutorial, we’ll guide you through the process of decoding a transaction hash on the Polygon Network and extracting its information. You don’t need to be a tech expert to follow along – we’ll be using user-friendly tools like web3.js, abi-decoder, node, and JavaScript. So, whether you’re a beginner or a seasoned developer, let’s dive into the world of blockchain and uncover the power of web3.js together!
Similar to our last tutorial, lets start by setting up our environment.
First, lets create our directory:
Mkdir decodeTest
cd decodeTest
Now, run the following to install the latest web3 Packages
npm i web3
Next, create a file inside the directory called index.js
touch index.js
Just like the last article, we’ll use the index.js to be our launch pad for this application. To continue, open up your index.js file in your favorite editor to get started.
At the top of you’re index.js file, lets add the lines to require web3:
npm i web3
Next, we need to connect to the Polygon network. We can do this by adding the following lines to our index.js file to create a new instance of the web3 object and setting the provider to an Infura endpoint for the Polygon network. Keep in mind, you’ll need an infura id to complete this next step. You can obtain this by going to infura.io and signing up. A basic account is free and set-up only takes a few minutes. Once this is done and you’ve obtained your key, update you file with the lines below:
const Web3 = require('web3');
const web3 = new Web3('https://polygon.infura.io/v3/YOUR-PROJECT-ID');
Now, we can use the web3.eth.getTransaction() method to retrieve the transaction details for the transaction hash we want to decode. If you read our previous article on capturing event transactions, you may have noticed that you receive the transaction hash in the event objects that you received. You can use this transaction hash in the next section, or you can hunt down a transaction hash on your own on polygonscan.
web3.eth.getTransaction('0x...', function(error, result){
if(!error){
console.log(result);
} else {
console.error(error);
}
});
The returned object will contain various properties such as the to, from, value, and input fields. We can access these individual values using their parameter names. For example, if the ABI of the contract includes a parameter named “value”, we can access the value of this parameter by calling result.value. In this case, since our value is in wei, we can also convert this value by passing the value into th eweb3.utils.fromWei() method to convert the value of the transaction from Wei to Ether. If you’d like, you can do this by adding the following code to your result:
const etherValue = web3.utils.fromWei(result.value, 'ether');
console.log(etherValue);
For most transaction responses, there’s also an Input field that contains some additional encoded data from the transaction and is returned in hexadecimal format. In the next step, we’ll go further and decode this data so we can see what’s inside.
5. In order to decode the Input data, we’re going to add an additional library to make things easier. Return to your console and type in the following:
npm i abi-decoder
This should install the decoder libraries to our project. Now, add the below code to your index.js file:
const abiDecoder = require('abi-decoder');
We’ll also want to add a reference to our contract ABI and add it to our decoder. Lets add this above our getTransaction call. Somewhere near the top of the file.
Const contractABI = [ABI CONTRACT];
abiDecoder.addABI(contractABI);
You’ll wan tot replace the ABI contract above with the actual ABI contract from the contract you’re attempting to decode. Finding this is actually pretty simple. First, go to Polygon scan and type the hex value of your contract address in the search bar as shown below:
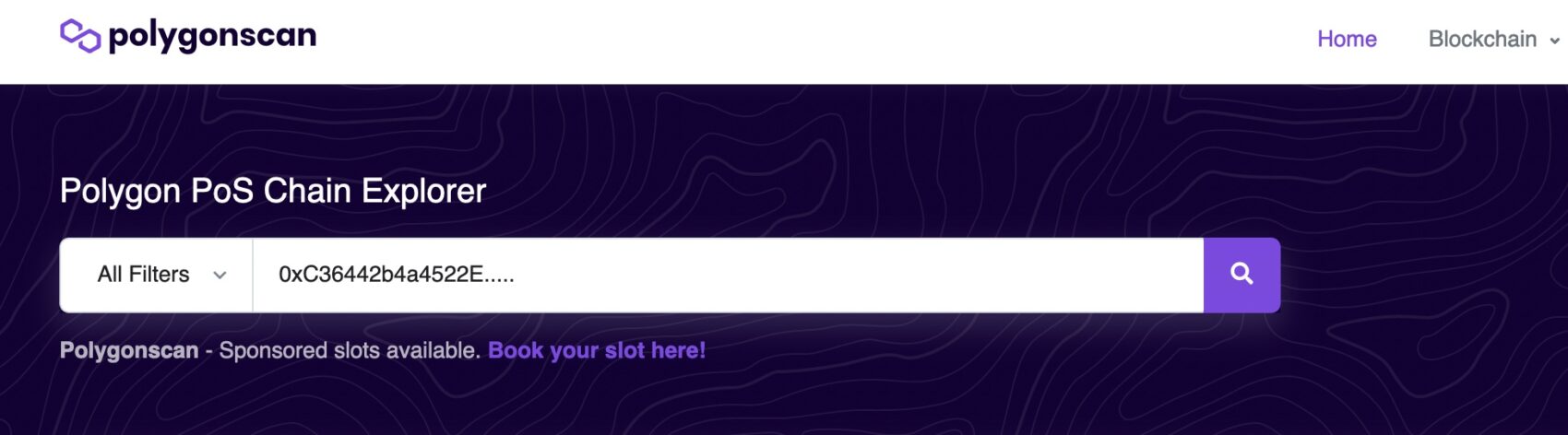
Next, look for the “Contract” tab about half way down the page. This will display info on your contract:
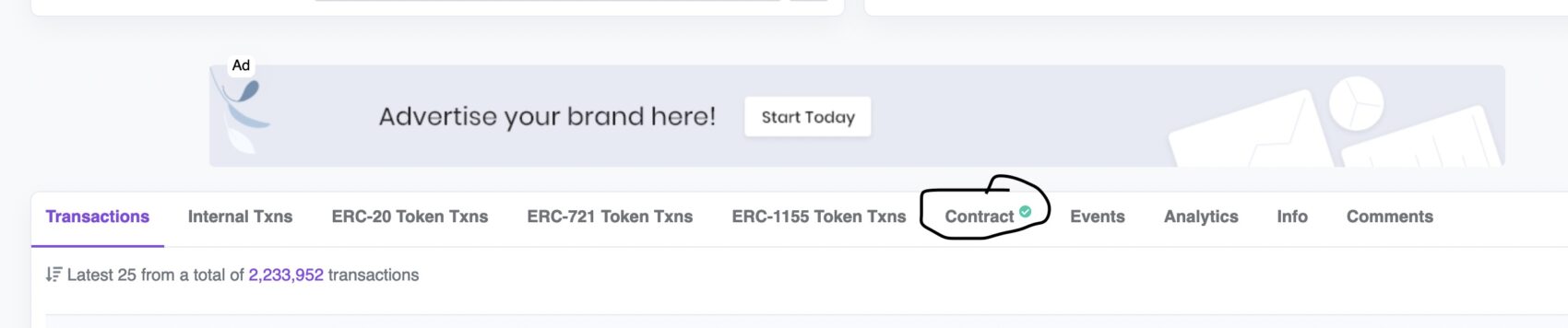
Finally, scroll down until you see the “ABI” section. This is the info you need for the ABI info above. Lets copy it and add it as and replace the section marked “[ABI CONTRACT]” in the code snippet above.
Const contractABI = [ABI CONTRACT]; <----- REPLACE
Now, inside the getTransaction response, we’re going to pass in the input parameter from the response to our decoder and get the actual value. Then we’ll log that value out to our console.
const decodedData = abiDecoder.decodeMethod(result.input);
console.log(decodedData);
In the above example, the abiDecoder.decodeMethod()
method returns an object that contains the decoded data, with each parameter in the ABI mapped to its corresponding value in the transaction. If everything worked right, you should see some info pertaining to the transaction. There are some cases though that this data won’t decode correctly, so watch out for errors or null’s coming back, if something went wrong.
Using web3.js and JavaScript to decode a hexadecimal transaction hash on the Polygon network is a relatively simple process. By connecting to the network, retrieving the transaction details, and decoding the data using the ABI of the contract, developers can gain valuable insight into the information stored on the blockchain. With this knowledge, developers can build more powerful and sophisticated applications on top of the Polygon network.