“As a follow up post to our MRTK2 Unity Development Setup, we’ll explore building and deploying a simple Hololens app in Unity.”
Overview
In this post, we are going to build a simple MRTK2 Unity app for Microsoft Hololens. Previously, we added all the items we needed to be able to build a Mixed Reality application for the Hololens. Now that we are setup, let’s go ahead and start building!
If you are coming from the video, you can find the SlideHandler.cs script in the scripts section below.
Setting up MRTK2 in the Unity app
First, create a new blank Unity Project where we will setup the Mixed Reality Toolkit 2 (MRTK2). Next, go to the MRTK2 Releases page and download the Microsoft.MixedReality.Toolkit.Unity.Foundation.2.1.0.unitypackage package. You can optionally install the examples, extensions, and tools packages, but those are not required. Once downloaded, double click it and import everything into your blank Unity Project. After this completes, you may get a message asking you to “Apply Mixed Reality Toolkit Default Settings”. Click Apply, and Reload the scene if prompted.
Now you should see a Mixed Reality Toolkit menu tab in Unity. Click it and select Add to Scene and Configure. A dialog will pop up with some Mixed Reality Profile options. Pick the configuration profile that matches your Hololens version. Viola! We are ready to set up Virtual Reality support for our Unity app using MRTK2.
Add Virtual Reality Support
Before we start building, we need to tell the Hololens to process our app using Mixed Reality. To do this, simply go to Edit -> Project Settings -> Player and open the section for XR Settings. Check the Virtual Reality Supported and under the Virtual Reality SDKs section, click the plus and add Windows Mixed Reality.
Holographic Remoting Player Setup
First, let’s create a simple cube and test it using the Holographic Remoting Player we installed in the previous post. Add a Cube to the scene and set its position 0, 0, 5, and rotation to 45, 45, 45. Next, under the Window menu, go to XR -> Holographic Emulation. If you get a warning, you probably need to setup Virtual Reality support, see the section above. Select Remote to Device for the Emulation mode and your version of Hololens device.
Now, you will need to open up the Holographic Remoting Player app on your Hololens that we installed in our earlier blog post. (Link here if you need it). Once opened, you should see a dark screen with the IP address of your Hololens. Enter this IP address into Remote Machine section in Unity and click Connect. Now, enter play mode and put on your Hololens and you should see the cube you created! Play around with editing its scale and position in Unity and notice how it updates in your Hololens in realtime.
Setting Up Our MRTK2 Unity App Scene
To quickly set up our MRTK2 Unity app scene, the MRTK2 gives us access to a lot of prefabs. In your Project view, navigate to Assets -> MixedRealityToolkit.SDK -> Features -> UX -> Interactable -> Prefabs. You’ll find lots of goodies for both Hololens 1 and 2. In our case, we’re going to search the project for “PinchSlider” and drag the prefab into the scene. Next, Set its position to 0, 0, 2.
Now, lets make our slider do something. We’ll make it control the color of our cube. In the scripts section below, you can find the SlideHandler.cs script. Add this script to your project and then add it to your Cube component. Once added, drag the PinchSlider gameobject onto the SlideHandler script’s Pinch Slider section. Now, select the PinchSlider gameobject and click the plus to add an On Value Change method. Drag the Cube object in and select the SlideHandler.OnSlideChange method to run when the PinchSlider’s value is changed.
Finally, we are ready to test! Use Holographic Emulation to test the app on the Hololens in Play Mode. As you drag the slider left, the cube should turn black and as you drag it right, it should turn white.
Building the MRTK2 Unity App
To start building our app with Unity, go to File -> Build Settings. Make sure to click the Universal Windows Platform, then click Switch Platform. Next, click Add Open Scene, to add our Scene to the build. Your settings should look similar to the following:
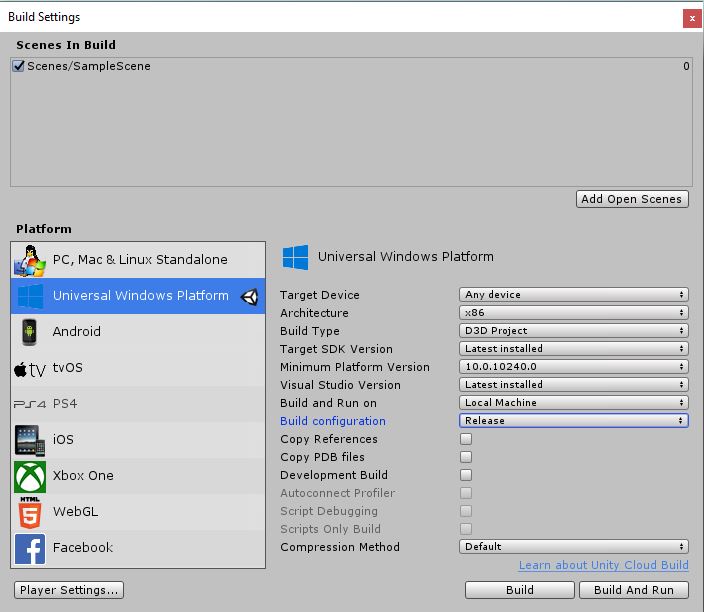
Then click Build, create a new Build folder and select it to start the build. We will handle deploying to our Hololens device using USB through Visual Studio 2019, after Unity completes its build. Note, if you do not see any options for Universal Windows Platform, then you’ll need to install it. Here is a link with directions from our previous post.
If you have build errors make sure you have all the correct dependencies outlined in our previous post. Most notably, check that under Target SDK Version there is 10.0.18362.0.
Deploying to Hololens Using Visual Studio 2019
Once the Unity build is complete, navigate to the Build folder you created in the previous step and open it. Inside, you should see a Visual Studio solution that Unity created. Make sure you open it with Visual Studio 2019 if you have multiple versions. It may prompt you to install missing tools. Go ahead and install the tools it recommends.
Next, we are going to deploy our app onto our Hololens through USB, so plug your Hololens into your computer. Once it is plugged in, go to the top bar and set the build type to Debug, the architecture to x86, and the deploy mode to Device. Then go to Debug -> Start Debugging (Or click the Device button with the green arrow). The project should start building and deploying to your Hololens. Note, if you don’t have the Device option available, go to Project -> Set as StartUp Project.
While deploying, Visual Studio might ask you for a device pin. On your Hololens, go to Settings -> Update & Security -> For Developers. Then under Device Discovery, click Pair. A code will appear. Enter this into the Visual Studio dialog box to continue the deployment.
Conclusion
Congratulations on your first simple Hololens deploy! Visual Studio lets you pick Debug, Release, or Master deploys. Debug runs a debugger and a profiler. Release has just the profiler, and Master has neither and is meant for production builds. Once the app is on your Hololens, you can open it from there without needing to run it through Visual Studio.
If you’d like to remove the MRTK2 diagnostics panel from your build, you can go into your Unity Scene, click on MixedRealityToolkit gameobject, and under the script click Copy & Customize, then create a clone of the Default Profile. Once you clone it, go back onto the MixedRealityToolkit script and uncheck Enable Diagnostics System.
MRTK2 Unity App Scripts
// SlideHandler.cs
using UnityEngine;
using Microsoft.MixedReality.Toolkit.UI;
public class SlideHandler : MonoBehaviour
{
public PinchSlider pinchSlider;
MeshRenderer meshRenderer;
// Start is called before the first frame update
void Start()
{
meshRenderer = GetComponent<MeshRenderer>();
}
public void OnSlideChange()
{
if (meshRenderer != null)
{
float rgbValue = pinchSlider.SliderValue;
meshRenderer.material.color = new Color(rgbValue, rgbValue, rgbValue);
}
}
}